Raw String Literals in C# 11 .NET 7
Raw string literals are a new feature in C# 11 .NET 7, which may appear to be a mess at first but may have some good reasons to be used. However, there may be some valid reasons for using it.
Lets see this in action and see why we would use these
Step 1:
Open Visual Studio 2022. For this demonstration, I’m using Visual Studio 2022, version 17.4.3; if you want.NET 7 support in Visual Studio, use this version or higher.
Select Create a new project.
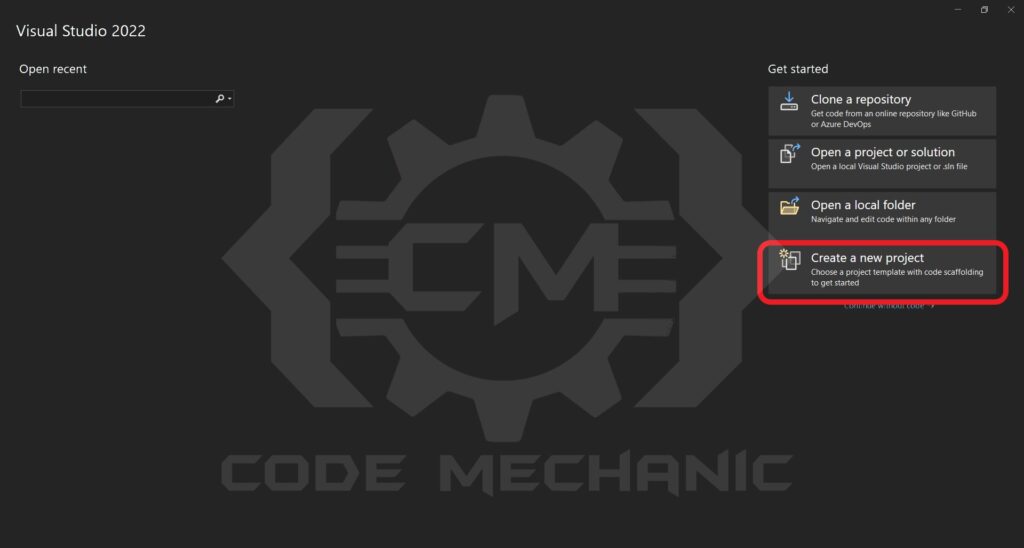
Step 2
Search Console App
Select Console App (make sure you choose the Console App not Console App .NET Framework as it is a older version of .NET)
Click “Next.”
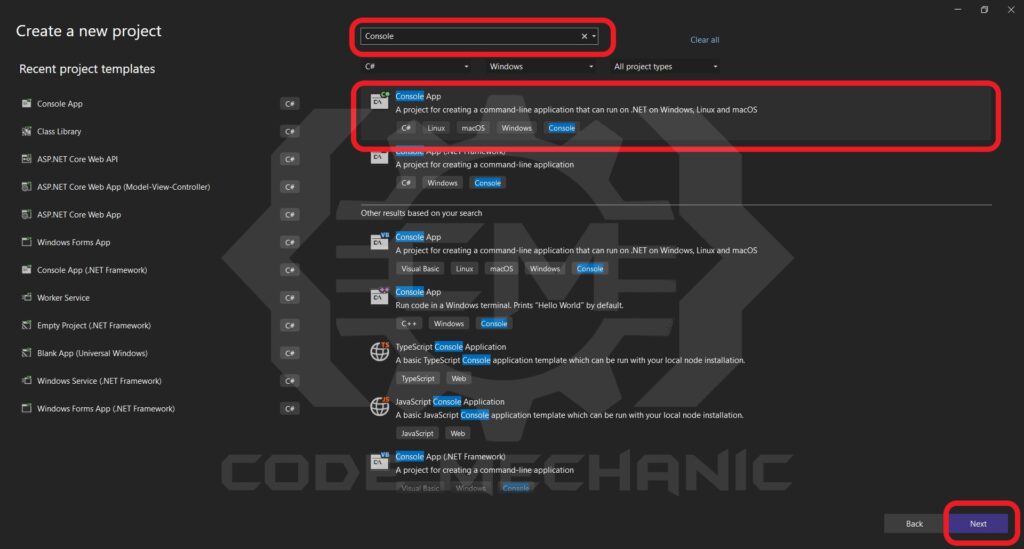
Step 3
Provide the name in the project name field.
Click “Next.”
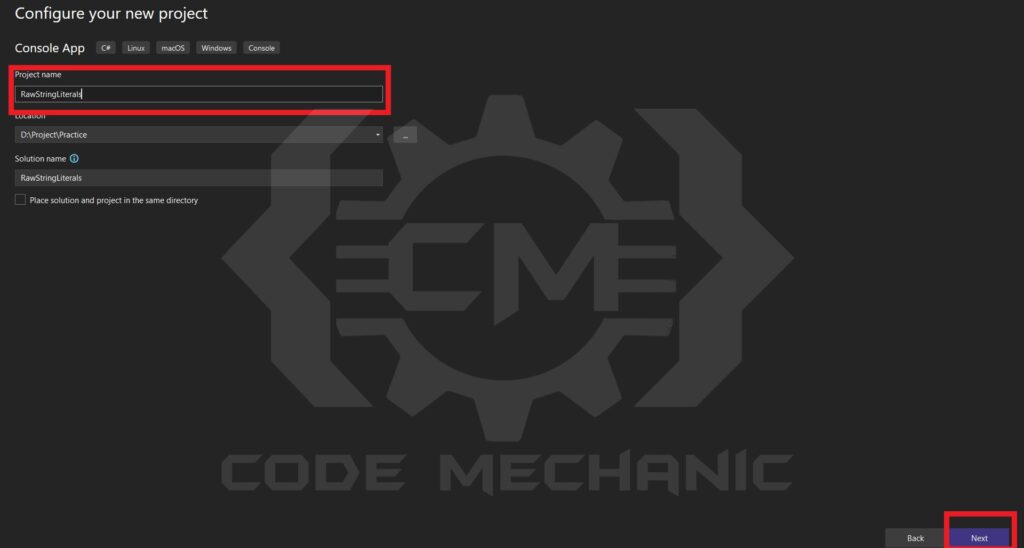
Step 4
In this step, you have to select the framework.
Select .NET 7.0
Click “Next.”
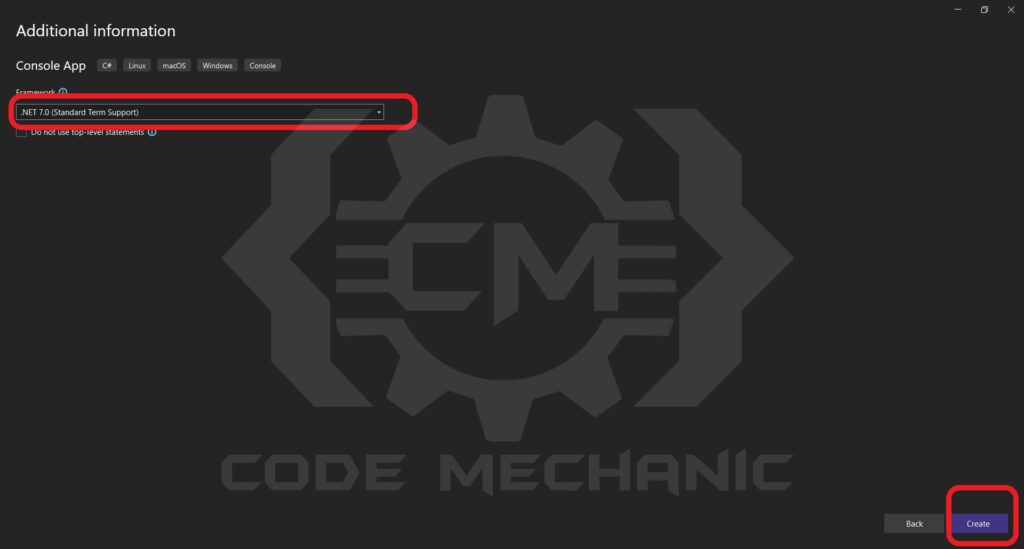
Step 5
Let’s say we have string variable demoString
and we use verbatim character to make a demo string
string demoString = @"C:\NewFolder";
We can assign whatever we want in the demoString using verbatim character, notice that i have declare path and it doesn’t need escape character.
We can even use string interpolation very easily with verbatim character like I have used in the below string example.
string filename = "test.txt";
string demoString = @$"C:\NewFolder\{filename}";
However, there is a problem with this approach. Let’s say I want to paste JSON code in here and then print it on screen. Let’s create demostring with JSON String
string demoString = @"{
""id"": 0,
""userName"": ""string"",
""password"": ""string"",
""isActive "": true,
""roleId "": 0,
""employeeUniqueId "": ""string"",
""locationIds "": ""string"",
""companyId "": 0,
""emailId "": ""string""
}";
Did you notice something? We have two double quotes for every double quote in the JSON string, which makes it look a little sloppy, but if we run this, the result will be correct.
Notice that single quotes in the output which it had originally
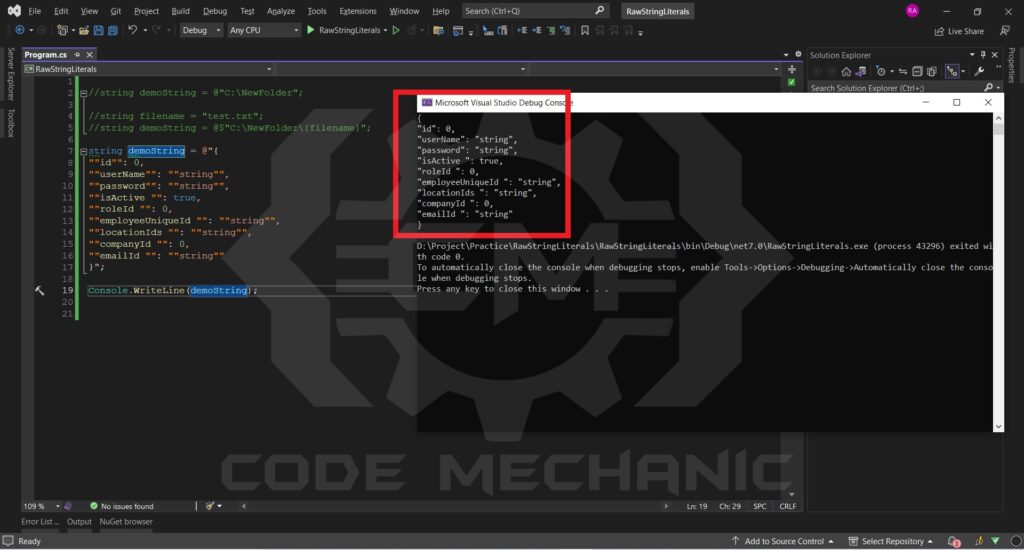
It looks right in the output, but in the code it does not look good, and if you change the indentation of the string, it gets messy with the JSON format.
Now lets use raw string literals to solve this problem next
You have to start with 3 double quotes in a row to start raw string literal
i.e. “””YOUR STRING”””
Let’s copy and paste the above JSON into the three double quotes in a row
string demoString = """
{
"id": 0,
"userName": "string",
"password": "string",
"isActive": true,
"roleId": 0,
"employeeUniqueId": "string",
"locationIds": "string",
"companyId": 0,
"emailId": "string"
}
""";
You can see that there is only one double quote here, and it is also indented.
Let me show you the output also
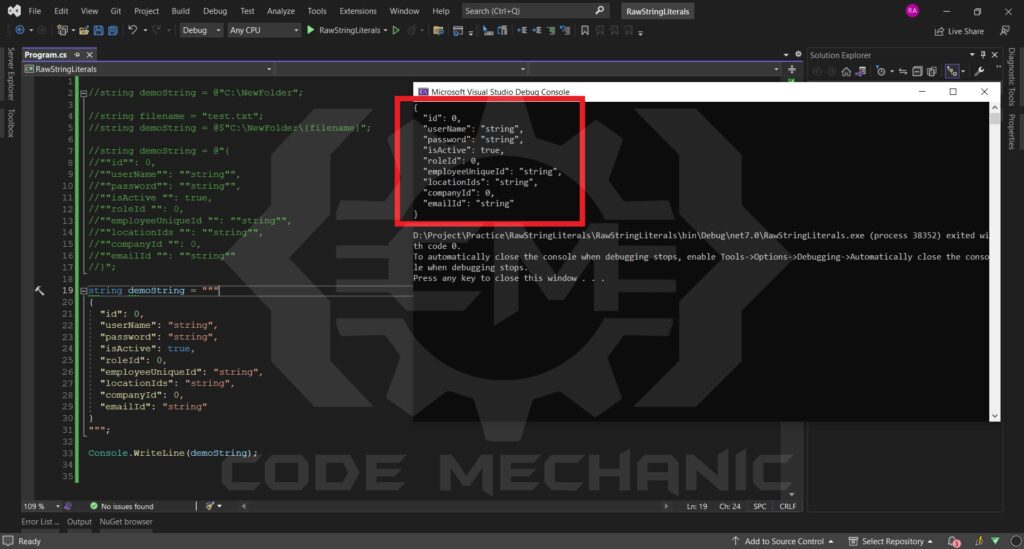
You can notice that it is on left and well indented and only have single double quotes both in output and in the code.
Now we have a better solution. you might think it is weird to write string with 3 double quotes, honestly i also think so but there are benefits also doing so this way
The most significant advantage is that whatever you paste between quotes will function exactly as you have entered it.
If you have a requirement that includes 3 double quotes in between the JSON string mentioned above in the example, you can simply add 1 more double quote at either end of the string, resulting in 4 double quotes at the end of the string. let me show you by example code
string demoString = """"
{
"id": 0,
"userName": """string",
"password": "string",
"isActive": true,
"roleId": 0,
"employeeUniqueId": "string",
"locationIds": "string",
"companyId": 0,
"emailId": "string"
}
"""";
Now lets run this and see the output
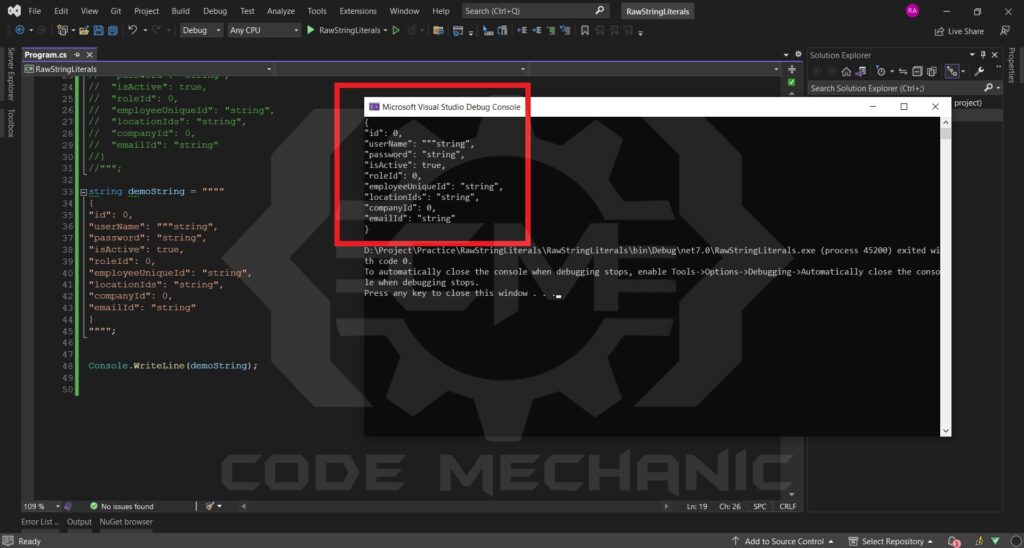
In the output you can see the 3 quotes in the username value
You could actually make this as long as you want string with 100 double quotes also
Now you might require to use string interpolation along with this we have
to put value of username variable into the demostring JSON string username value but how?
string username = "admin";
string demoString = $"""
{
"id": 0,
"userName": {username},
"password": "string",
"isActive": true,
"roleId": 0,
"employeeUniqueId": "string",
"locationIds": "string",
"companyId": 0,
"emailId": "string"
}
""";
This code creates issue because in string interpolation
We use { braces to place values so to solve this problem
We have very simple solution we have to write double $$ in front of demo string and 2 opening and closing curly braces
string username = "admin";
string demoString = $$"""
{
"id": 0,
"userName": { { username} },
"password": "string",
"isActive": true,
"roleId": 0,
"employeeUniqueId": "string",
"locationIds": "string",
"companyId": 0,
"emailId": "string"
}
""";
And now it works perfect let me show you the output
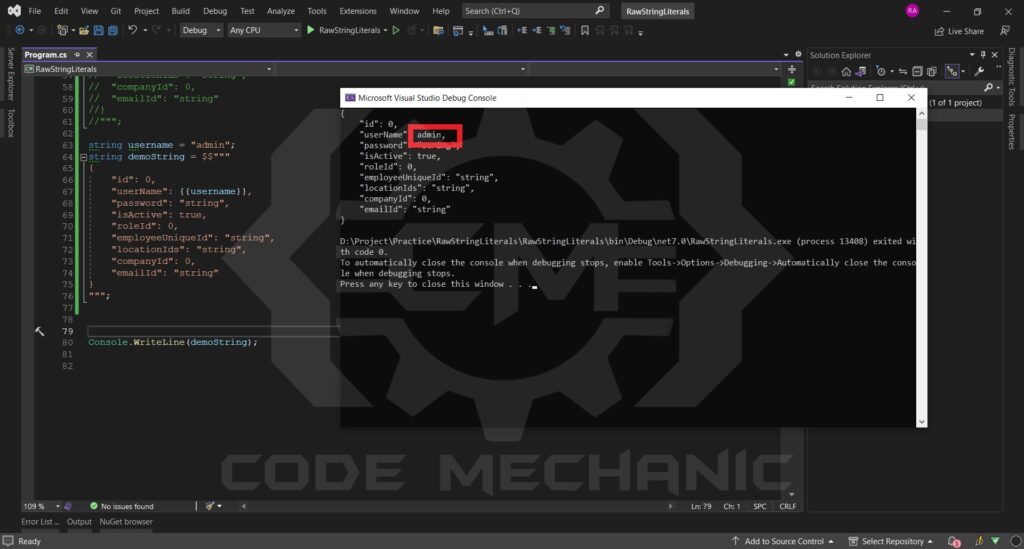
I know it might not look good but it may work great in some scenarios, but just take a note of this feature that this is available now you can use it wherever you find it relevant.
And yes you have to keep in mind that during creating raw string literal you have to start with new line instead of starting with the same line with 3 double quotes.
string demoString = """{
"id": 0,
"userName": "string",
"password": "string",
"isActive": true,
"roleId": 0,
"employeeUniqueId": "string",
"locationIds": "string",
"companyId": 0,
"emailId": "string"
}""";
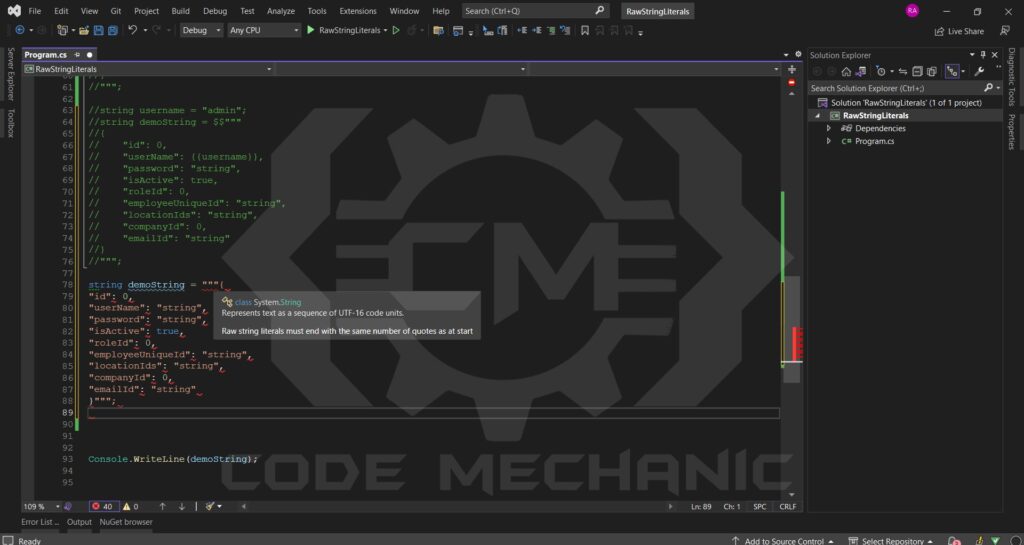
this code will give you error “Raw String Literals must end with the same number of quotes as at start”.
So this is the new feature in C# 11. That might look messy, but it gives you another option, and it is good to have an option. Options allow us to do things differently.
If you like this article also have a look @ .NET 7 Overview article where you can find the Changes and Support Cycle of it.
Thanks for reading the article. Please let me know your thoughts about this feature. Do let me know where you are going to use this feature in the comment section or mail me @ [email protected].